Migrating to MapKit: A New Chapter for Unlimited App
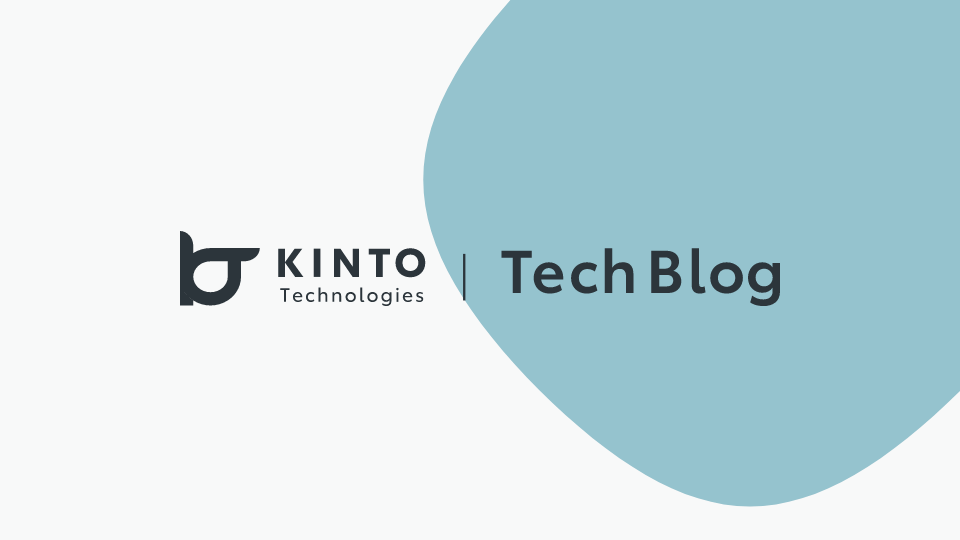
This aricle is the entry for day 19 in the KINTO Technologies Advent Calendar 2024 🎅🎄 Hello, I am GOSEO, an iOS engineer in the Mobile App Development Group at KINTO Technologies. Currently, I’m working on the app Unlimited. Interestingly, 66% of the iOS developers working on Unlimited are from overseas, and I enjoy chatting with them in English every day. I am also a fan of Paradox games and am currently hooked on Crusader Kings Ⅲ.
This article is the entry for day 19 in the [KINTO Technologies Advent Calendar 2024]
Evaluating the migration from Google Maps to MapKit in the Unlimited App
In recent years, improvements in Apple Map’s performance have sparked interest in transitioning from Google Maps to MapKit. This shift is expected to reduce usage fees and enhance app performance and user experience. In this article, I will walk you through the implementation process, the challenges we encountered, and the outcomes of migrating from Google Maps to MapKit in the Unlimited app.
Evaluating the migration from Google Maps to MapKit and its Process
1. Rendering maps and creating gradient lines
In the Unlimited app, gradient lines are rendered on Google Maps. To replicate this functionality in MapKit, we tested the use of MKGradientPolylineRenderer
. By setting colors and specifying the start and end points using locations
, we examined whether this implementation would work effectively. Additionally, I considered that this feature could be used in the future to dynamically change the line's color based on the user's speed exceeding the limit.
func mapView(_ mapView: MKMapView, rendererFor overlay: MKOverlay) -> MKOverlayRenderer {
if let polyline = overlay as? MKPolyline {
let gradientRenderer = MKGradientPolylineRenderer(polyline: polyline)
gradientRenderer.setColors(
[Asset.Colors.primary.color, Asset.Colors.cdtRouteGradientLight.color],
locations: [0.0, 1.0]
)
gradientRenderer.lineWidth = 2.0
return gradientRenderer
}
return MKOverlayRenderer(overlay: overlay)
}
2. Differences in tap detection
In Google Maps, detecting taps on the map or markers is straightforward. However, MapKit does not offer a built-in API for this functionality. To detect taps on the map itself, we used UITapGestureRecognizer
. For marker taps, we handled them using the didSelect
and didDeselect
methods. It was a bit challenging to figure out whether a tap was on the map or a marker, but we resolved this by checking if there was a marker at the tapped location. Challenge: Setting up custom gestures required extra effort, but we were able to confirm that it works as intended.
let tapGestureRecognizer = UITapGestureRecognizer(target: context.coordinator, action: #selector(context.coordinator.handleMapTap(_:)))
tapGestureRecognizer.delegate = context.coordinator
mapView.addGestureRecognizer(tapGestureRecognizer)
3. Adding and managing markers
On the Unlimited map, multiple types of markers often overlap. To handle this, we implemented a system using zPriority
to display markers in order of importance. By reusing instances of the same marker image, we avoided generating separate instances for each marker, which improved performance. Challenge: The default tap animation wouldn’t go away... After much trial and error, we found a solution. Instead of adding an image directly to MKAnnotationView, we
added a UIView as a subview of the annotation view, then added a UIImageView to the UIView, and finally set the image on the UIImageView
. This effectively disabled the default animation. The solution was truly a stroke of genius from one of my teammates!
4. Slow rendering
When we tested the map using driving data from Oita to Fukuoka, we found a bug where the line rendering couldn’t keep up during repeated zooming in and out after the map was displayed. The dataset contained 23,000 coordinate points, and rendering occurred every time the map view changed. This caused significant memory and CPU resource consumption during UI updates. Challenge: Rendering couldn’t keep up with large numbers of coordinate points. We tackled this by using the Ramer-Douglas-Peucker algorithm to simplify and reduce similar coordinate points. This allowed us to consolidate the data into a single polyline by simplifying and segmenting the polylines.
// A function to interpolate UIColor. Returns the color interpolated between two colors based on the fraction value
func interpolateColor(fraction: CGFloat) -> UIColor {
// Retrieve the RGBA components of the start and end colors
let fromComponents = Asset.Colors.primary.color.cgColor.components ?? [0, 0, 0, 1]
let toComponents = Asset.Colors.cdtRouteGradientLight.color.cgColor.components ?? [0, 0, 0, 1]
// Interpolate colors based on the fraction
let red = fromComponents[0] + (toComponents[0] - fromComponents[0]) * fraction
let green = fromComponents[1] + (toComponents[1] - fromComponents[1]) * fraction
let blue = fromComponents[2] + (toComponents[2] - fromComponents[2]) * fraction
return UIColor(red: red, green: green, blue: blue, alpha: 1)
}
// A function to generate polyline information from an array of coordinates
func makePolylines(_ coordinates: [CLLocationCoordinate2D]) -> [PolylineInfo] {
// Return an empty array If the coordinates array is empty
guard !coordinates.isEmpty else { return [] }
// Calculate the chunk size (at least the entire set as one chunk)
let chunkSize = coordinates.count / 20 > 0 ? coordinates.count / 20 : coordinates.count
var cumulativeDistance = 0.0
Let totalDistance = coordinates.totalDistance() // Calculate the total distance
var previousEndColor: UIColor = Asset.Colors.primary.color
var previousEndCoordinate: CLLocationCoordinate2D?
var polylines: [PolylineInfo] = []
// Divide the coordinates into chunks and process each chunk
let chunks = stride(from: 0, to: coordinates.count, by: chunkSize)
.map { startIndex -> [CLLocationCoordinate2D] in
// Retrieve the coordinates of the chunk and add the last coordinates from the previous chunk
var chunk = Array(coordinates[startIndex..<min(startIndex + chunkSize, coordinates.count)])
if let lastCoordinate = previousEndCoordinate {
chunk.insert(lastCoordinate, at: 0)
}
previousEndCoordinate = chunk.last
return chunk
}
for chunk in chunks {
Let chunkDistance = chunk.totalDistance() // Calculate the distance of the chunk
Let startFraction = cumulativeDistance / totalDistance // Calculate the fraction for the start point
cumulativeDistance += chunkDistance
Let endFraction = cumulativeDistance / totalDistance // Calculate the fraction for the end point
let startColor = previousEndColor
let endColor = interpolateColor(fraction: CGFloat(endFraction)) // Calculate end color using interpolation
previousEndColor = endColor
// Simplify the polyline (reduce points while maintaining high accuracy)
let simplified = PolylineSimplifier.simplifyPolyline(chunk, tolerance: 0.00001)
let polyline = MKPolyline(coordinates: simplified, count: simplified.count)
// Add the polyline information to the list
polylines.append(PolylineInfo(
polyline: polyline,
startFraction: startFraction,
endFraction: endFraction,
startColor: startColor,
endColor: endColor
))
}
return polylines
}
// A function to simplify coordinates (implements the Ramer-Douglas-Peucker algorithm)
static func simplifyPolyline(_ coordinates: [CLLocationCoordinate2D], tolerance: Double) -> [CLLocationCoordinate2D] {
// Return the coordinates as is if there are 2 or fewer points, or if the tolerance is less than 0
guard coordinates.count > 2 else { return coordinates }
guard tolerance >= 0 else { return coordinates }
var result: [CLLocationCoordinate2D] = []
var stack: [(startIndex: Int, endIndex: Int)] = [(0, coordinates.count - 1)]
var include: [Bool] = Array(repeating: false, count: coordinates.count)
include[0] = true
include[coordinates.count - 1] = true
// Process recursively using a stack
while !stack.isEmpty {
let (startIndex, endIndex) = stack.removeLast()
let start = coordinates[startIndex]
let end = coordinates[endIndex]
var maxDistance: Double = 0
var currentIndex: Int?
// Find the farthest point from the current line
for index in (startIndex + 1)..<endIndex {
let distance = perpendicularDistance(point: coordinates[index], lineStart: start, lineEnd: end)
if distance > maxDistance {
maxDistance = distance
currentIndex = index
}
}
// If the farthest point exceeds the tolerance, include it and subdivide further
if let currentIndex, maxDistance > tolerance {
include[currentIndex] = true
stack.append((startIndex, currentIndex))
stack.append((currentIndex, endIndex))
}
}
// Add only the coordinates where include is true to the result
for (index, shouldInclude) in include.enumerated() where shouldInclude {
result.append(coordinates[index])
}
return result
}
// A function to calculate the vertical distance between a point and a line
private static func perpendicularDistance(point: CLLocationCoordinate2D, lineStart: CLLocationCoordinate2D, lineEnd: CLLocationCoordinate2D) -> Double {
let x0 = point.latitude
let y0 = point.longitude
let x1 = lineStart.latitude
let y1 = lineStart.longitude
let x2 = lineEnd.latitude
let y2 = lineEnd.longitude
// Distance formula (distance between a point and a line in a 2D plane)
let numerator = abs((y2 - y1) * x0 - (x2 - x1) * y0 + x2 * y1 - y2 * x1)
let denominator = sqrt(pow(y2 - y1, 2) + pow(x2 - x1, 2))
// If the length of the line is 0, set the distance to 0
return denominator != 0 ? numerator / denominator : 0
}
5. Verification Results and Conclusions
We confirmed that similar functionality implemented with Google Maps in the Unlimited app can also be achieved using MapKit. This makes migration from Google Maps to Mapkit feasible. Additionally, this migration is likely to lower usage fees. Through this research, the Unlimited iOS team as a whole has gained a deeper understanding of MapKit’s capabilities.
Conclusion
Looking ahead, we plan to continue development using MapKit for this project. We will keep striving for further improvements to deliver even better services!
関連記事 | Related Posts
We are hiring!
【プロジェクトマネージャー】モバイルアプリ開発G/大阪
モバイルアプリ開発GについてKINTOテクノロジーズにおける、モバイルアプリ開発のスペシャリストが集まっているグループです。KINTOやmy routeなどのサービスを開発・運用しているグループと協調しながら品質の高いモバイルアプリを開発し、サービスの発展に貢献する事を目標としています。
【iOSエンジニア】モバイルアプリ開発G/東京
モバイルアプリ開発GについてKINTOテクノロジーズにおける、モバイルアプリ開発のスペシャリストが集まっているグループです。KINTOやmy routeなどのサービスを開発・運用しているグループと協調しながら品質の高いモバイルアプリを開発し、サービスの発展に貢献する事を目標としています。