Calling Generative AI (Azure OpenAI) with Java
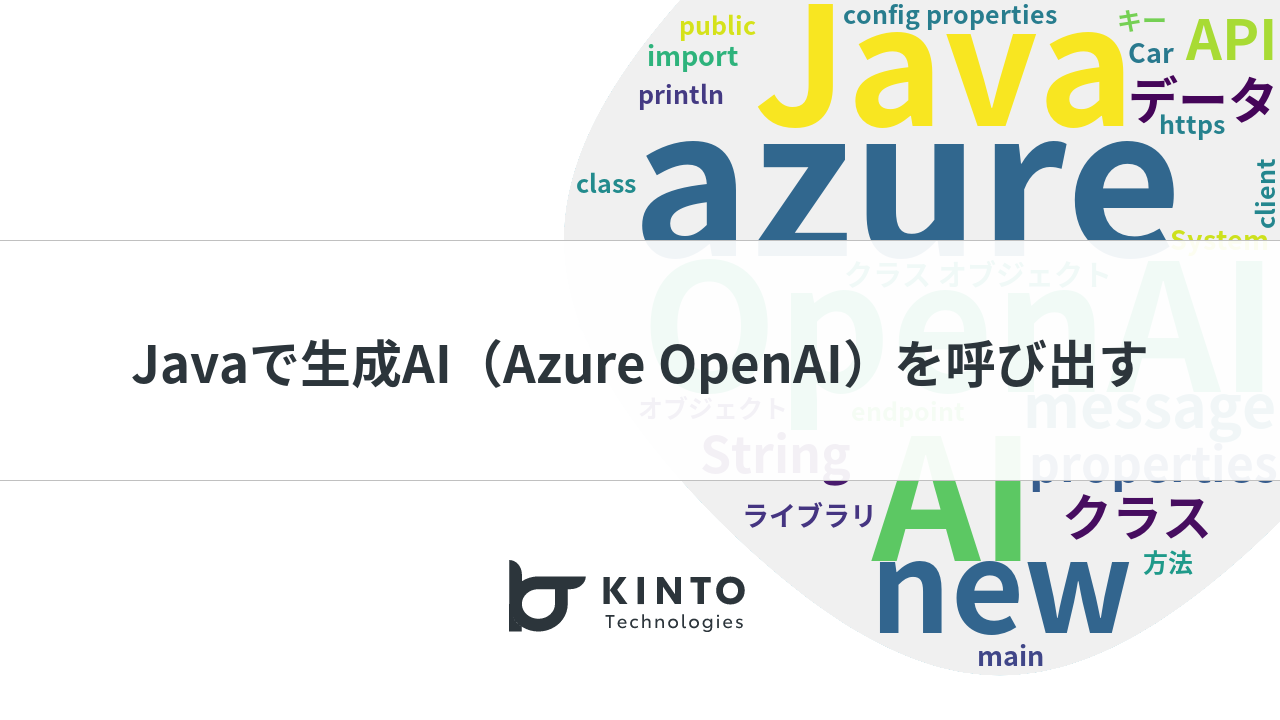
This article is the entry for day 9 in the KINTO Technologies Advent Calendar 2024.
Introduction
Hello, I'm Kameyama, an application engineer in the Digital Transformation Development Group.
In recent years, generative AI has been applied across different fields, and our development team is also working on building systems that makes the most of its capabilities.
Furthermore, since Java is widely used within our development team, we believed it would allow us to efficiently build an interface for generative AI while taking advantage of our existing knowledge and tools. With this in mind, this article will explain how to call generative AI using Java and process its results.
This article will cover the basic implementation of calling Azure OpenAI using Java, with simple code examples. Compared to OpenAI, Azure OpenAI is considered a platform with greater scalability and reliability, making it better suited for large-scale business systems.
Setting Up Azure Open AI
First, sign up for an Azure subscription:
Next, follow the instructions on the page below to obtain the endpoint and API key.
Access the Azure console here. *This page requires logging in with the account you registered earlier.
Setting Up the OpenAI Library
To call Azure OpenAI, the Azure SDK library will be used. This SDK library allows for simple and efficient coding to call generative AI in Azure OpenAI.
For Gradle:
dependencies {
implementation 'com.azure:azure-ai-openai:1.0.0-beta.12'
implementation 'com.azure:azure-core-http-okhttp:1.7.8'
}
For Maven:
<dependencies>
<dependency>
<groupId>com.azure</groupId>
<artifactId>azure-ai-openai</artifactId>
<version>1.0.0-beta.12</version>
</dependency>
<dependency>
<groupId>com.azure</groupId>
<artifactId>azure-core-http-okhttp</artifactId>
<version>1.7.8</version>
</dependency>
</dependencies>
The version used here is the latest available at the time of writing. Refer to the Official Documentation for the most up-to-date version.
In particular, the Azure OpenAI client library for Java that we will use this time is currently in beta, so we recommend that you use the stable version when it is released in the future.
Calling the Azure OpenAI Chat Model in Practice
Reference: https://github.com/Azure/azure-sdk-for-java/tree/main/sdk/openai/azure-ai-openai
/src/main/resource/config.properties
endpoint=https://{Resource Name}.openai.azure.com/
apiKey={API Key}
Enter the obtained Azure OpenAI endpoint and API key here to manage them in a separate file. You can manage sensitive information according to your own or your team's policies.
/src/main/java/com/sample/app/Main.java
Package com.sample.app; // match your package name
import com.azure.ai.openai.OpenAIClient;
import com.azure.ai.openai.OpenAIClientBuilder;
import com.azure.ai.openai.models.*;
import com.azure.core.credential.AzureKeyCredential;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Load the properties file *Modify as needed if using a different method to manage key information.
Properties = new Properties();
try (InputStream input = Main.class.getClassLoader().getResourceAsStream("config.properties")) {
if (input == null) {
System.out.println("config.properties File not found.") ;
return;
}
properties.load(input);
} catch (IOException ex) {
System.out.println(ex.getMessage());
return;
}
// Retrieve configuration values from properties
String endpoint = properties.getProperty("endpoint");
String apiKey = properties.getProperty("apiKey");
// Create OpenAI client
var client = new OpenAIClientBuilder()
.endpoint(endpoint)
.credential(new AzureKeyCredential(apiKey))
.buildClient();
// Prepare the prompt
List<ChatRequestMessage> messages = new ArrayList<>()
.setTemperature(0.7) // Response randomness, the higher more diverse (0.0~2.0)
.setMaxTokens(100) // Maximum number of response tokens
.setFrequencyPenalty(0.0) // Penalty for frequently occurring words (-2.0~2.0)
.setPresencePenalty(0.6); // Penalty for things relates to existing topics (-2.0~2.0)
messages.add(new ChatRequestSystemMessage("You are an excellent AI assistant."));
messages.add(new ChatRequestUserMessage("For beginners, please explain the difference between classes and objects in Java."));
// Set the request option
var options = new ChatCompletionsOptions(messages);
var chatCompletions = client.getChatCompletions("gpt-4o", options); //Specify the deployment name or generative AI model name to use
// Send the request and retrieve the result
for (ChatChoice choice : chatCompletions.getChoices()) {
ChatResponseMessage message = choice.getMessage();
System.out.printf("Index: %d, Chat Role: %s.%n", choice.getIndex(), message.getRole());
System.out.println("Message:");
System.out.println(message.getContent());
}
}
}
When calling the generation AI, you can set various parameters. These are parameters that cannot currently be set in the ChatGPT application you normally use, and being able to adjust these parameters is one of the benefits of calling the generation AI from a program. In this case, we set four parameters (temperature, maxTokens, frequencyPenalty, and presencePenalty), but there are many other parameters. See here for more details.
In addition, the following two types of messages were set in the messages section. The former ChatRequestSystemMessage can be executed without setting.
-
ChatRequestSystemMessage
This message is used to set the behavior and roles of the generative AI model, defining the tone of the conversation and response style.
-
ChatRequestUserMessage
This message is used to convey specific questions or instructions from the user to the AI, and the response is returned from OpenAI as the return value.
For the first argument of getChatCompletions, enter a deployment name or a model name. The deployment name can be obtained from the Azure portal. If using OpenAI outside of Azure, enter a model name "gpt-4o" or "gpt-3.5-turbo," etc. (In the example above, the model name is entered.)
.gitignore
/src/main/resources/config.properties
If managing it as explained in this article with /src/main/resource/config.properties, add the above line to .gitignore.
Especially when managing it in a repository, be sure to handle sensitive information such as API keys with utmost care.
Execution Result
We were able to get the following response from OpenAI. (The actual response is in Markdown format.)
Index: 0, Chat Role: assistant.
Message:
The difference between classes and objects in Java is an important concept for beginners learning programming. Here's an easy-to-understand explanation:Class
- Blueprint: You can think of a class as a blueprint or template for creating an object. It defines the attributes (fields) and behaviors (methods) of an object.
- Declaration: In Java, the
class
keyword is used to define a class. For example, a class representing a car can be defined as follows:public class Car { // Field (attribute) String color; int year; // Method (behavior) void drive() { System.out.println("The car is driving"); } }
Object
- Instance: An object is an entity (instance) generated from a class. It holds specific data and can perform operations on that data.
- Creation: In Java, the
new
keyword is used to create an object from a class. For example, to create an object from theCar
class:public class Main { public static void main(String[] args) { // Create an instance of the Car class Car myCar = new Car(); myCar.color = "Red"; myCar.year = 2020; // Call the object's method myCar.drive(); } }
Summary
- A class is a template for creating objects, defining their attributes and behaviors.
- An object is an actual instance of the class, holding specific data and executing the defined behaviors.
By understanding this basic relationship, you can begin to build more complex programs.
Conclusion
This article provided a basic introduction to using Azure OpenAI with Java.
Since there is still limited information on integrating OpenAI with Java, I hope this guide will be useful to you.
Next time, I'd like to explore more advanced methods, so stay tuned!
関連記事 | Related Posts
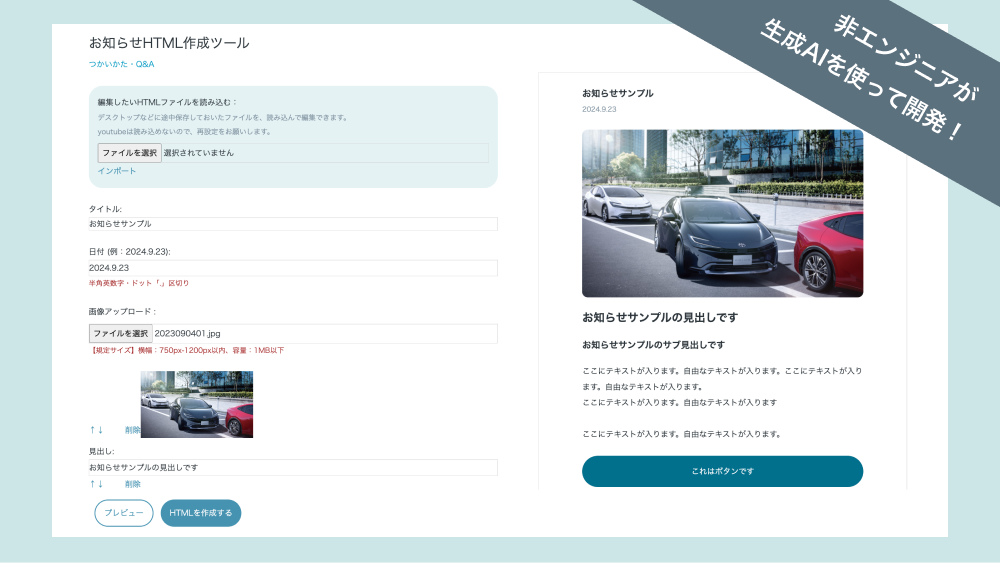
Generative AI and Copilot — How a Non-Engineer like Me Developed an Operation Tool Using AI
![Cover Image for [生成AI][Copilot] 非エンジニアの私がAIを使って運用ツールを開発した話](/assets/blog/authors/yamayuki/01.png)
[生成AI][Copilot] 非エンジニアの私がAIを使って運用ツールを開発した話
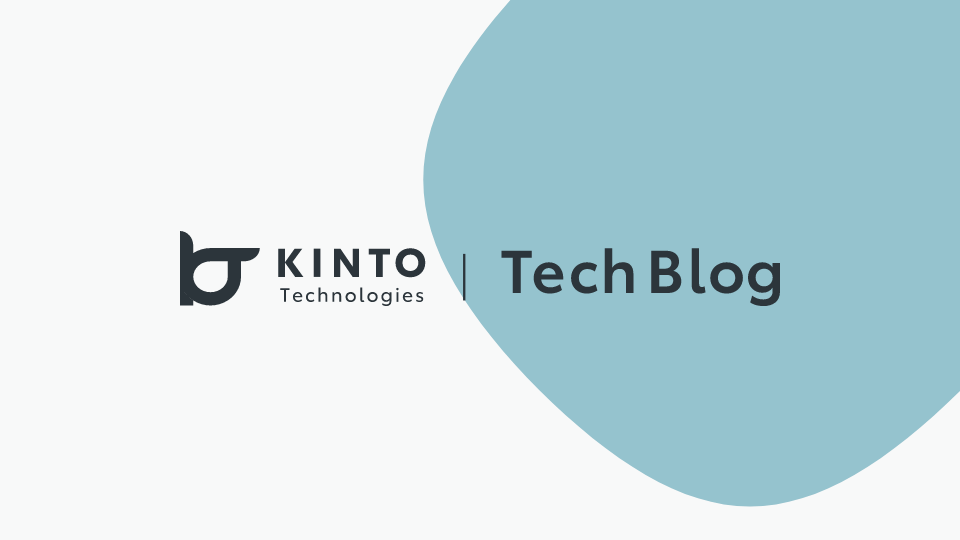
Rapid Verification: Generative AI-Powered Chat API for MVP Development
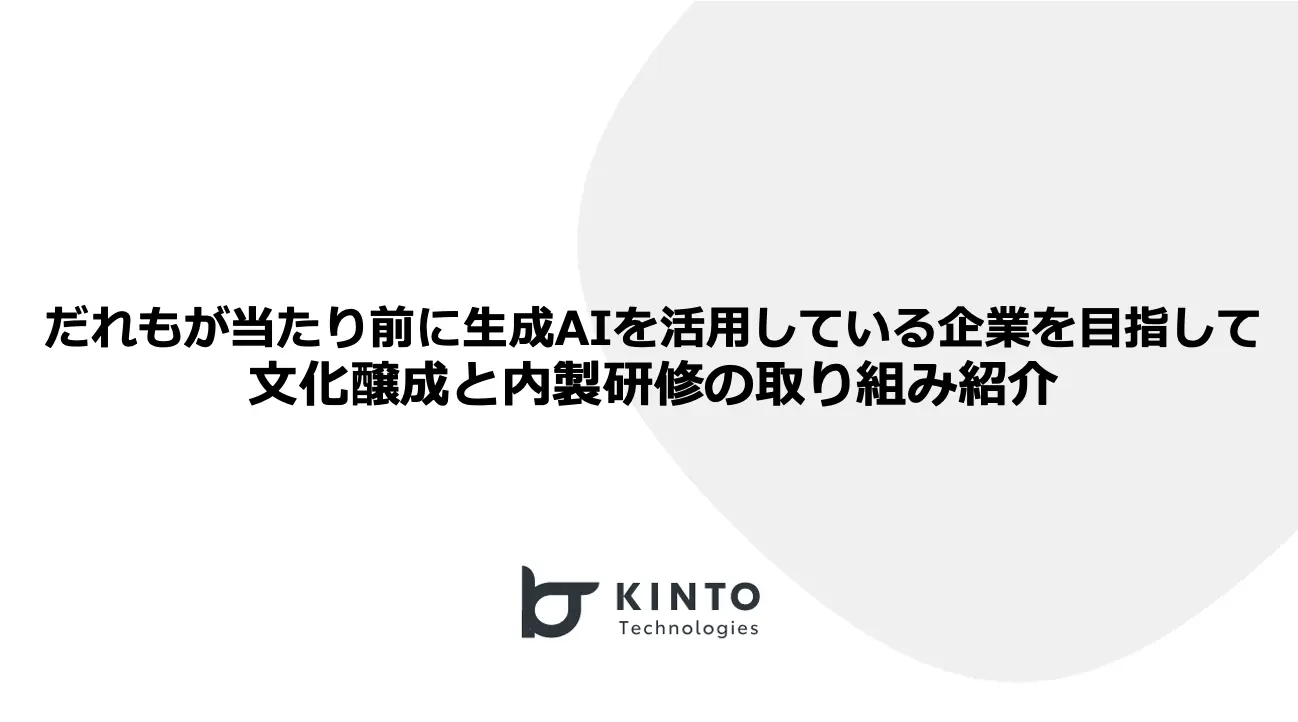
"Aiming to Be a Company Where Generative AI is Second Nature" – Culture Building and In-House Training Initiatives
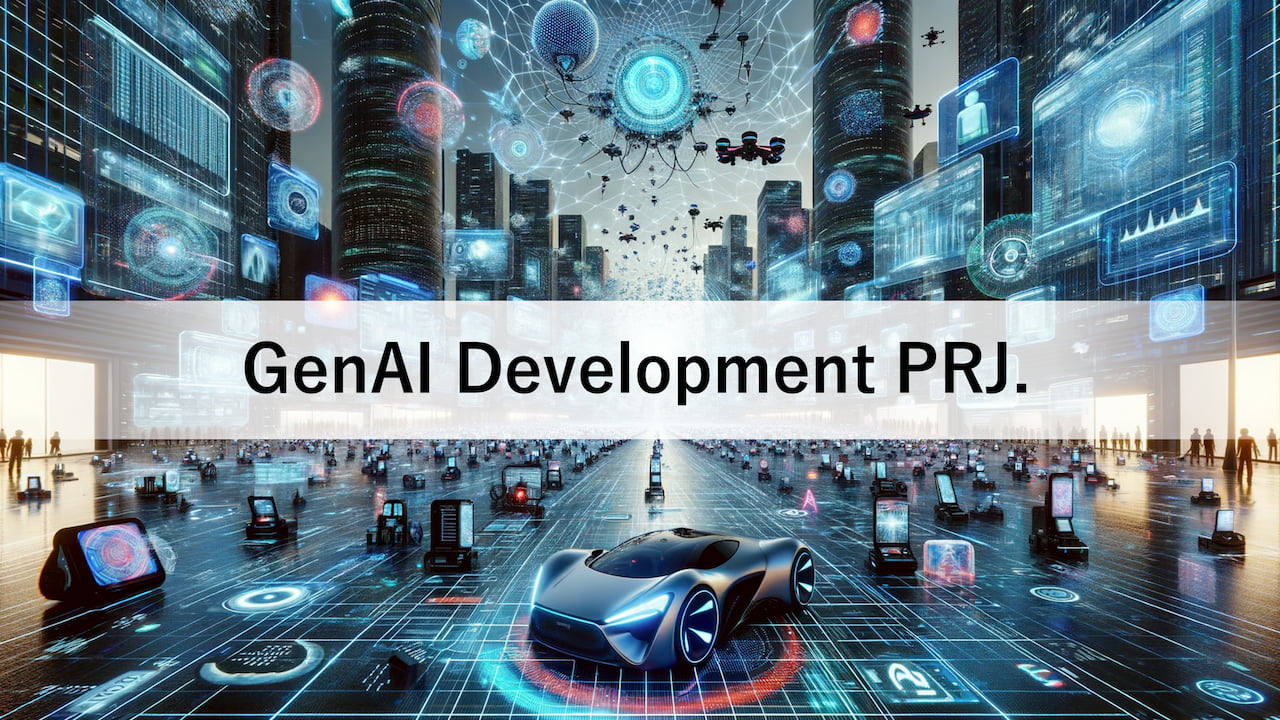
Introduction to our Generative AI Development Project
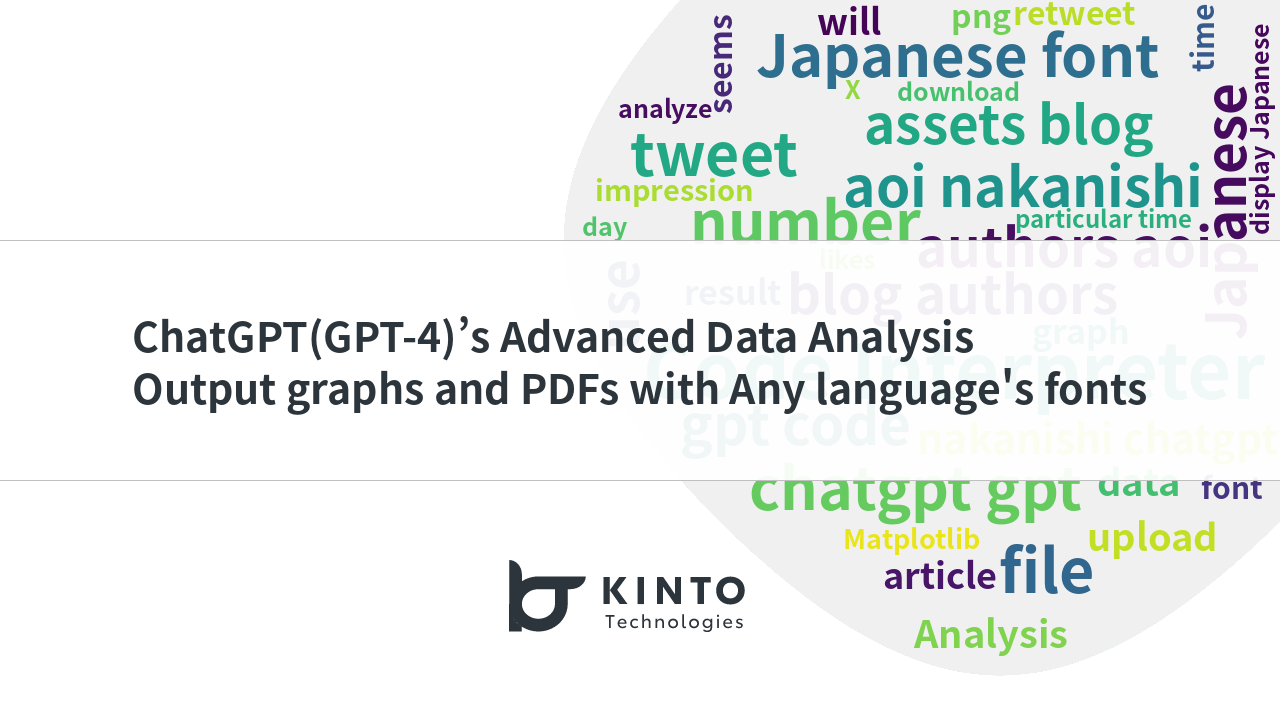
ChatGPT (GPT-4) Advanced Data Analysis (formerly Code Interpreter), how to output graphs, images, and PDF files with Japanese fonts to analyze X (formerly Twitter)
We are hiring!
生成AIエンジニア/生成AI活用PJT/東京・名古屋・大阪
生成AI活用PJTについて生成AIの活用を通じて、KINTO及びKINTOテクノロジーズへ事業貢献することをミッションに2024年1月に新設されたプロジェクトチームです。生成AI技術は生まれて日が浅く、その技術を業務活用する仕事には定説がありません。
機械学習エンジニア(レコメンドシステム)/マーケティングプロダクトG/東京
マーケティングプロダクトグループについてKINTOサービスサイト内で、パーソナライズ/ターゲティング/レコメンドなどのWEB接客系プロダクトを企画、開発、分析まで一貫して担当しています。そのほか、おでかけスポットをAIで提案するアプリ『Prism Japan』を開発・運営しています。