Introduction to TypeScript
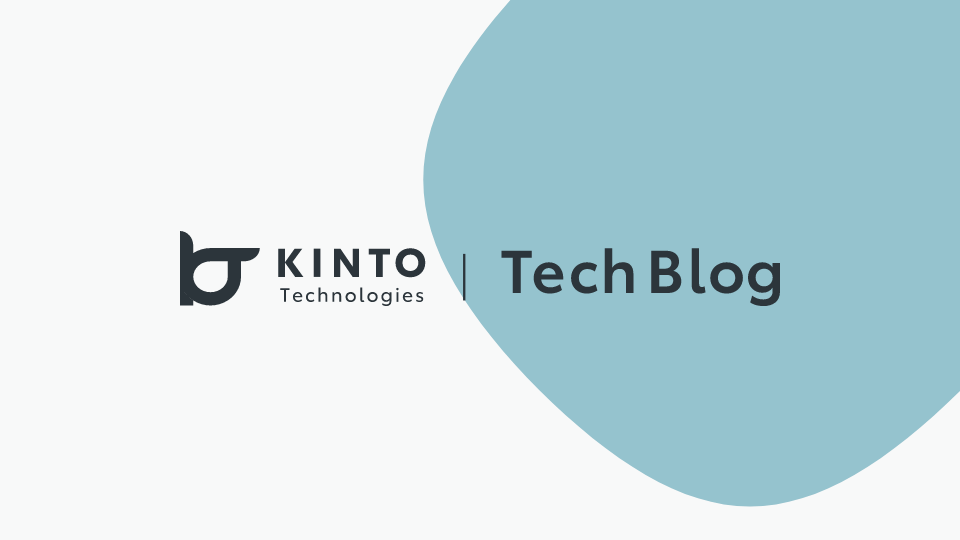
Introduction
Hello! My name is Ren.M from KINTO Technologies' Project Promotion Group. My main role is to develop the front-end of KINTO ONE (Used Vehicles). This time, we'd like to introduce the basics of TypeScript, specifically the type definitions.
Target audience of this article
- Those who want to learn about TypeScript type definitions
- Those who want to learn TypeScript following their JavaScript studies
What is TypeScript?
TypeScript is a language that operates as an extension of JavaScript, so both TypeScript and JavaScript use the same syntax. In traditional JavaScript, there's no obligation to specify data types, allowing for more flexible coding. However, improving program reliability and mitigate issues such as inconsistent typing was required so we sought a solution. Enter TypeScript, leveraging static typing to address these concerns. By understanding type definitions you will be able to code smoothly and ensure safer data transfer.
How TypeScript differs from JavaScript
JavaScript allows for different assignments of the following data types:
let value = 1;
value = "Hello";
However, in TypeScript, the behavior is as follows:
let value = 1;
// It is not a number type so it cannot be assigned
value = "Hello";
// Can be assigned because it is the same number type
value = 2;
The main type of data
// string type
const name: string = "Taro";
// number type
const age: number = 1;
// boolean type
const flg: boolean = true;
// array string type
const array: string[] = ["apple", "banana", "grape"];
Explicitly defining a type after :
is called a type annotation.
Type inference
As mentioned above, TypeScript automatically assigns types without using type annotations. This is called type inference.
let name = "Taro"; // string type
// Bad: name cannot be assigned as it is a type of string
name = 1;
// Good: Can be assigned because it is a type of string
name = "Ken";
Array definition type
// an array that accepts only a number type
const arrayA: number[] = [1, 2, 3];
// an array that accepts only number or string types
const arrayB: (number | string)[] = [1, 2, "Foobar"];
interface
The type definition of an object can be an interface .
interface PROFILE {
name: string;
age?: number;
}
const personA: PROFILE = {
name: "Taro",
age: 22
};
Similar to the age
mentioned above, with a "?" following the key elementyou can also make the property arbitrary through a grant.
// 'age' element is not required
const personB: PROFILE = {
name: "Kenji",
};
Intersection Types
The concatenation of multiple types is called Intersection Types. The following applies to STAFF
.
type PROFILE = {
name: string;
age: number;
};
type JOB = {
office: string;
category: string;
};
type STAFF = PROFILE & JOB;
const personA: STAFF = {
name: "Jiro",
age: 29
office: "Tokyo",
category: "Engineer",
};
Union Types
More than one type can be defined using | (pipe).
let value: string | null = "text";
// Good
value = "kinto";
// Good
value = null;
// Bad
value = 1;
In the event of arrays
let arrayUni: (number | null)[];
// Good
arrayUni = [1, 2, null];
// Bad
arrayUni = [1, 2, "kinto"];
Literal Types
Assignable values can also be explicitly typed.
let fruits: "apple" | "banana" | "grape";
// Good
fruits = "apple";
// Bad
fruits = "melon";
typeof
If you want to inherit a type from a declared variable, use typeof.
let message: string = "Hello";
// inherits the string type from the message
let newMessage: typeof message = "Hello World";
// Bad
newMessage = 1;
keyof
keyof is used to create a type from the property name (key) from the type of an object.
type KEYS = {
first: string;
second: string;
};
let value: keyof KEYS;
// Good
value = "first";
value = "second";
// Bad
value = "third";
enum
Enum (enumeration type) is a function that automatically assigns a consecutive number. The following assigns 0 to SOCCER
and 1 to BASEBALL
. enum improves readability and makes maintenance easier.
enum SPORTS {
SOCCER,
BASEBALL,
}
interface STUDENT {
name: string;
club: SPORTS;
}
// 1 is assigned to club
const studentA: STUDENT = {
name: "Ken",
club: SPORTS.BASEBALL,
};
Generics
By using Generics, you can declare a type each time you use it. This is useful for situations where you want to repeat the same code in different types. Conventionally, T
and similar syntax are often used.
interface GEN<T> {
msg: T;
}
// declare the type of T when used
const genA: GEN<string> = { msg: "Hello" };
const genB: GEN<number> = { msg: 2 };
// Bad
const genC: GEN<number> = { msg: "message" };
When you define a default type, declarations such as <string>
are optional.
interface GEN<T = string> {
msg: T;
}
const genA: GEN = { msg: "Hello" };
You can also use extends
together to restrict the types that can be used.
interface GEN<T extends string | number> {
msg: T;
}
// Good
const genA: GEN<string> = { msg: "Hello" };
// Good
const genB: GEN<number> = { msg: 2 };
// Bad
const genC: GEN<boolean> = { msg: true }:
For use with functions
function func<T>(value: T) {
return value;
}
func<string>("Hello");
// <number> is not required
func(1);
// multiple types are allowed
func<string | null>(null);
In the event that extends
is used in a function
function func<T extends string>(value: T) {
return value;
}
// Good
func<string>("Hello");
// Bad
func<number>(123);
When used in conjunction with the interface
interface Props {
name: string;
}
function func<T extends Props>(value: T) {
return value;
}
// Good
func({ name: "Taro" });
// Bad
func({ name: 123 });
Conclusion
In this article, we covered the fundamentals of TypeScript. What are your thoughts? TypeScript's usage in front-end development has been on the rise, and I believe that learning and using TypeScript can prevent data type inconsistencies and facilitate safer development with fewer bugs. I hope this article was helpful! There are many other articles on the tech blog, so please check them out!
関連記事 | Related Posts
We are hiring!
【フロントエンドエンジニア】新車サブスク開発G/東京
新車サブスク開発グループについてTOYOTAのクルマのサブスクリプションサービスである『 KINTO ONE 』のWebサイトの開発、運用をしています。業務内容トヨタグループの金融、モビリティサービスの内製開発組織である同社にて、自社サービスである、TOYOTAのクルマのサブスクリプションサービス『KINTO ONE』のWebサイトの開発、運用を行っていただきます。
【フロントエンドエンジニア(コンテンツ開発)】新車サブスク開発G/東京
新車サブスク開発グループについてTOYOTAのクルマのサブスクリプションサービスである『 KINTO ONE 』のWebサイトの開発、運用をしています。業務内容トヨタグループの金融、モビリティサービスの内製開発組織である同社にて、自社サービスである、TOYOTAのクルマのサブスクリプションサービス『KINTO ONE』のWebサイトの開発、運用を行っていただきます。