Relearning JavaScript: Key Concepts of Scope
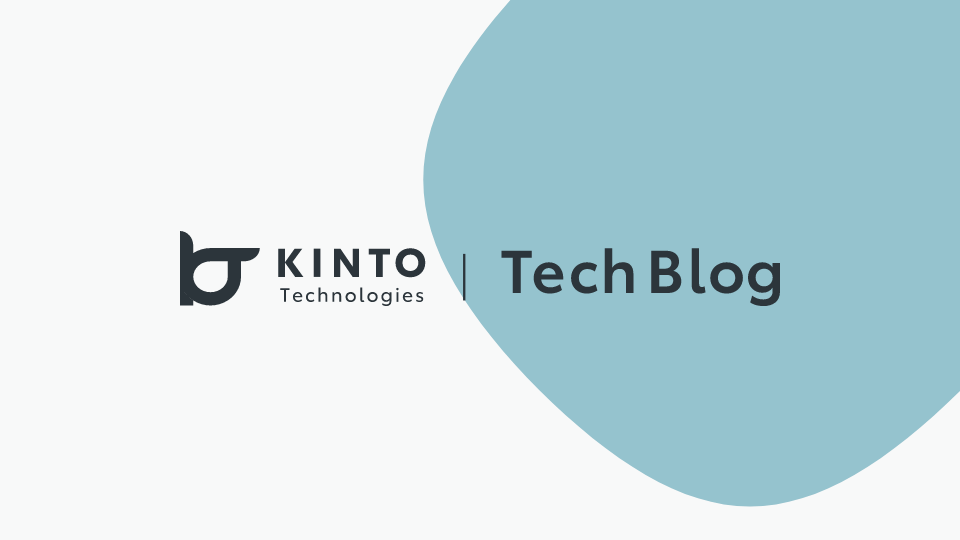
Introduction
Hello, and thank you for visiting. My name is ITOYU and I am in charge of front-end development in the new car subscription development group of the KINTO ONE Development Department.
Nowadays, it is common to use frameworks such as Vue.js, React, and Angular when creating web applications. The New Car Subscription Development Group is also using React and Next.js for development.
Libraries and frameworks are frequently updated, such as the release of React version 19 and Next.js version 15. Each time that happens, you need to get up to speed with the new features and changes, and update your knowledge.
In addition, the evolution of front-end technology has been remarkable in recent years. Libraries and frameworks that were in use until a few months ago become obsolete in a matter of months, and it is not uncommon for new libraries and frameworks to appear either.
With these being the circumstances, front-end developers need to be on constant lookout out for new technologies, libraries, and frameworks, gather information, and keep on learning.
This is the rule of front-end development, and also the joy of front-end development.
With passion and insatiable curiosity, front-end developers seek to learn and master new technologies, libraries, and frameworks to improve their skills, develop better web applications efficiently, pursue best practices, and become front-end gurus.
However, at the root of the libraries and frameworks in a front end, there is JavaScript. Do we really understand JavaScript 100% and use it with total mastery? Is it really possible to master libraries and frameworks without fully understanding JavaScript's core features? Can we really call ourselves front-end gurus?
Personally, I cannot confidently answer “yes” to that question.
So, in order to become a front-end expert, I decided to relearn JavaScript and fill in the gaps in my knowledge.
The purpose of this article
As a first step, goal is to learn about the basic JavaScript concept of Scope and understand it more deeply.
You may think this is too basic! I'm sure most front-end engineers use scope without even thinking about it.
However, when it comes to putting the concept of scope and the knowledge and names related to it into words, it is surprisingly difficult to do.
This article aims to provide a better understanding of the types of scopes to help you understand the concept of scope.
Reading it will most likely not furnish you with any new implementation methods. However, understanding the concept of scope should help you understand how JavaScript behaves, and lay the foundations for writing better code.
Scope
In JavaScript, there is a concept called Scope. Scope is the range within which variables and functions can be referenced by running code.
First, let us take a look at the following types of scope:
- Global scope
- Function scope
- Block scope
- Module scope
Global scope
Global scope refers to the scope that can be accessed from anywhere within the program.
The way to make a variable or function have global scope is roughly as follows:
- Variables added to the properties of the global object
- Variables that have script scope
Variables added to the properties of the global object
You can give variables and functions global scope by adding them to the properties of the global object.
The global object differs depending on the environment; in a browser environment it is the window object, and in a Node.js environment it is the global object.
In this example, I will assume you are coding for a browser environment, and show you how to add properties to the “window” object.
The way to do it is to declare variables and functions with “var.” Variables and functions declared with “var” get added as properties of the global object, and can be referenced from anywhere.
// A variable added to the properties of the “window” object
var name = 'KINTO';
console.log(window.name); // KINTO
You can also omit the window object when calling variables added to the global object.
// Calling a variable by omitting the “window” object
var name = 'KINTO';
console.log(name); // KINTO
Script-scoped variables
Script scope is the scope in which variables and functions declared at the top level of a JavaScript file or at the top level of a script element can be accessed.
Variables and functions declared at the top level with “let” or “const” will have script scope.
<! -- Variables that have script scope -->
<script>
let name = 'KINTO';
const company = ’KINTO Technologies Corporation’;
console.log(name); // KINTO
console.log(company); // KINTO Technologies Corporation
</script>
Top level
“Top level” means outside any functions or blocks.
This explanation of top-level declarations may seem confusing, so let's take a look at the following examples to see the difference between variables declared at the top level and those that are not:
<!-- Variables that have been declared at the top level -->
<script>
let name = 'KINTO';
const company = ’KINTO Technologies Corporation’;
console.log(name); // KINTO
console.log(company); // KINTO Technologies Corporation
</script>
<! -- Variables that have not been declared at the top level -->
<script>
const getCompany = function() {
const name = 'KINTO';
console.log(name); // KINTO
return name;
}
console.log(name); // ReferenceError: name is not defined
if (true) {
const company = ’KINTO Technologies Corporation’;
console.log(company); // KINTO Technologies Corporation
}
console.log(company); // ReferenceError: company is not defined
</script>
In the code above, the variable name
declared in the function getCompany
and the variable company
declared in the if
statement can only be referenced from within the function itself or the “if” statement’s block.
Differences between the global object and script scope
Variables declared at the top level with “let” or “const” will have global scope and can be referenced anywhere, just like ones declared with “var.”
However, unlike variables declared with “var,” ones declared with “let” or “const” do not get added to the properties of the global object.
// Variables declared with “let” or “const” do not get added to the properties of the global object
let name = 'KINTO';
const company = ’KINTO Technologies Corporation’;
console.log(window.name); // undefined
console.log(window.company); // undefined
Function scope
As mentioned in the previous example of variables without script scope, variables and functions declared within curly brackets {} within a function can only be referenced within that function. This is called ** function scope **.
const getCompany = function() {
const name = 'KINTO';
console.log(name); // KINTO
return name;
}
console.log(name); // ReferenceError: name is not defined
Since the variable “name” is declared inside the function “getCompany,” it can only be referenced inside that function. So if you try to reference the name variable from outside the function, an error will occur.
Block scope
The example above showing variables that do not have script scope also featured variables and functions declared within a range enclosed by curly brackets. These can only be referenced within that block. This is called block scope.
if (true) {
let name = 'KINTO';
const company = ’KINTO Technologies Corporation’;
console.log(name); // KINTO
console.log(company); // KINTO Technologies Corporation
}
console.log(name); // ReferenceError: name is not defined
console.log(company); // ReferenceError: company is not defined
Variables declared with “let” or “const” like this will have block scope. Variables declared inside curly brackets can only be referenced inside the curly brackets.
Module scope
Module scope is the referenceable scope of variables and functions declared inside a module. This means that variables and functions inside a module can only be accessed within that module, and cannot be referenced directly from outside it.
In order to reference variables or functions declared inside a module from outside it, you need to expose them to the outside using export
, and import them into the file using import
.
For example, I will declare some variables in the file module.js
as follows:
// module.js
export const name = 'KINTO';
export const company = ’KINTO Technologies Corporation’;
const category = ’subscription service’; // This variable has not been exported, so it cannot be referenced from outside.
The exported variables can be referenced by importing them into other files.
// Calling variables with module scope
import { name, company } from './module.js';
console.log(name); // Output: KINTO
console.log(company); // Output: KINTO Technologies Corporation
// This line causes an error because `category` has not been exported.
console.log(category); // ReferenceError: category is not defined
Trying to reference a variable that has not been exported will generate an error. This is because the module scope is hiding the variable from the outside.
// Calling a variable that does not have module scope
import { category } from './module.js'; // SyntaxError: The requested module './module.js' does not provide an export named 'category'
console.log(category); // The import fails, so this line cannot be run.
This shows how understanding module scope is extremely important for managing dependencies between modules in JavaScript.
Summary
- Scope is the range within which variables and functions can be referenced by running code.
- Global scope is the scope that can be referenced from anywhere.
- Script scope is the referenceable scope of variables and functions declared at the top level of either a JavaScript file or “script” element.
- Function scope is the referenceable scope of variables and functions declared inside curly brackets enclosed within a function.
- Block scope is the referenceable scope of variables and functions declared within a range enclosed by curly brackets.
- Module scope is the scope that is only referenceable inside a module.
This time, we explored the different types of scope in JavaScript. In the next article, I'll introduce additional concepts related to scope.
関連記事 | Related Posts
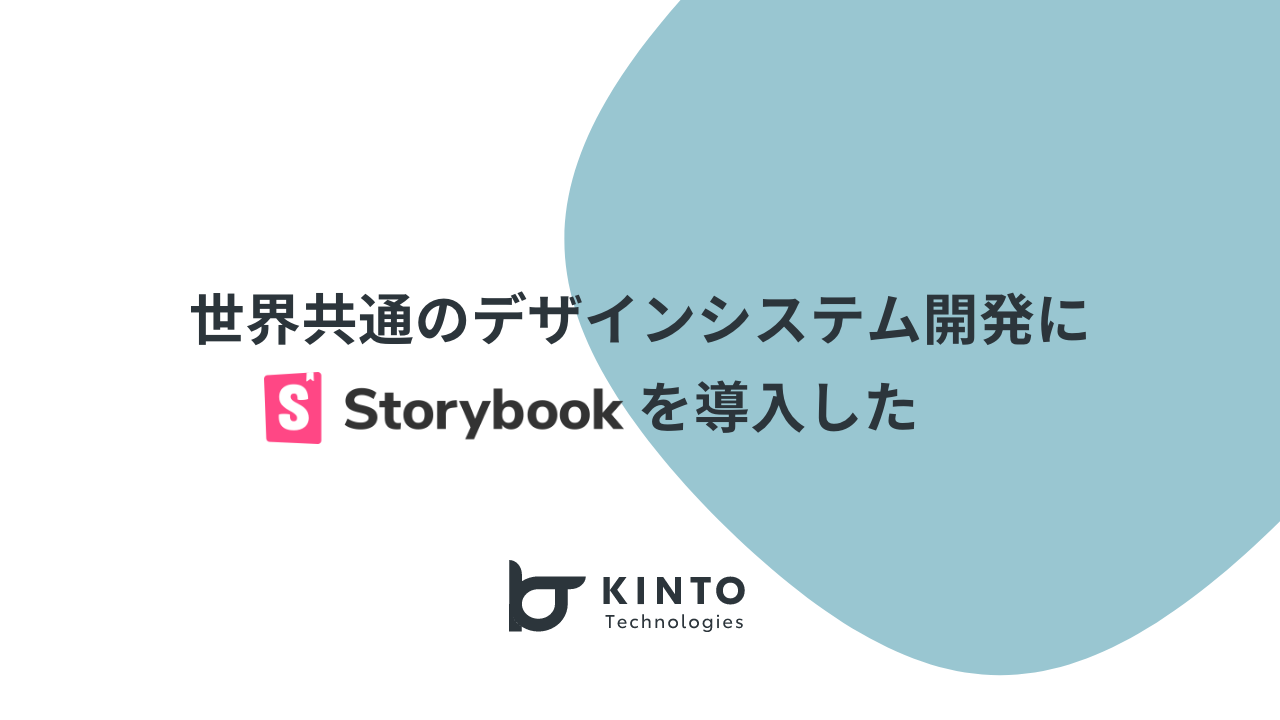
Introducing Storybook for the Development of a Universal Design System
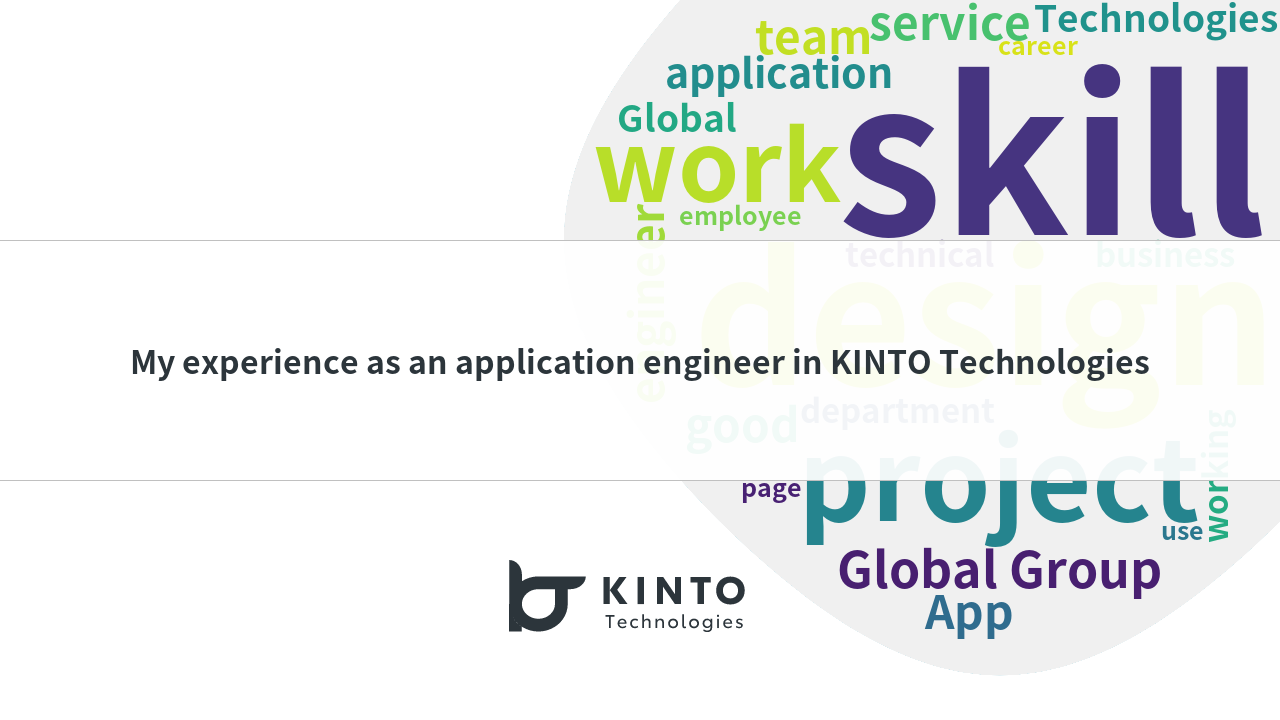
My experience as an application engineer in KINTO Technologies
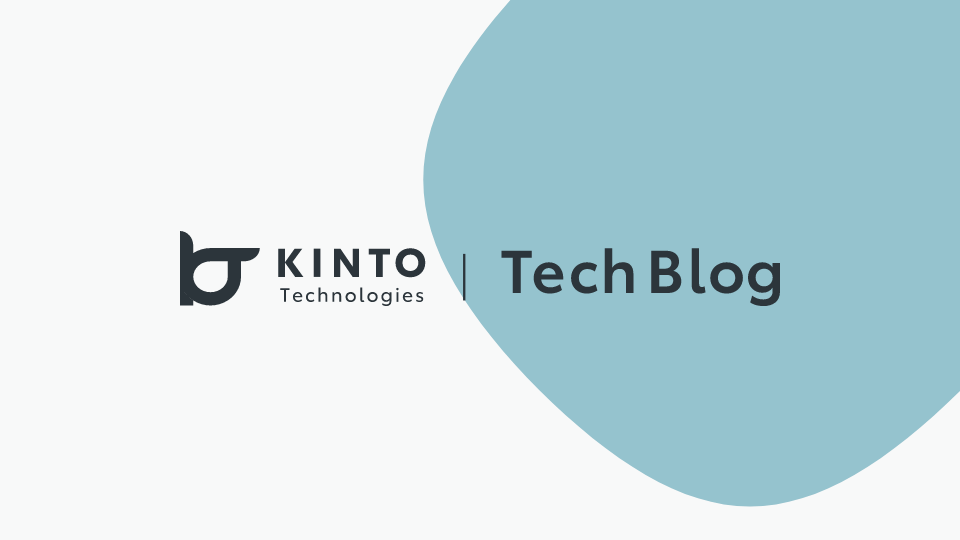
Introduction to TypeScript
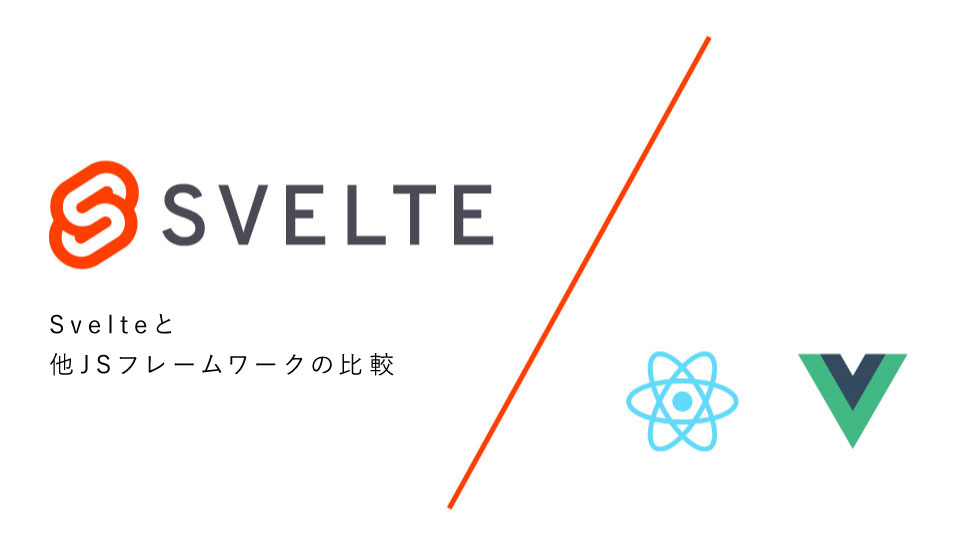
Insights from using SvelteKit + Svelte for a year
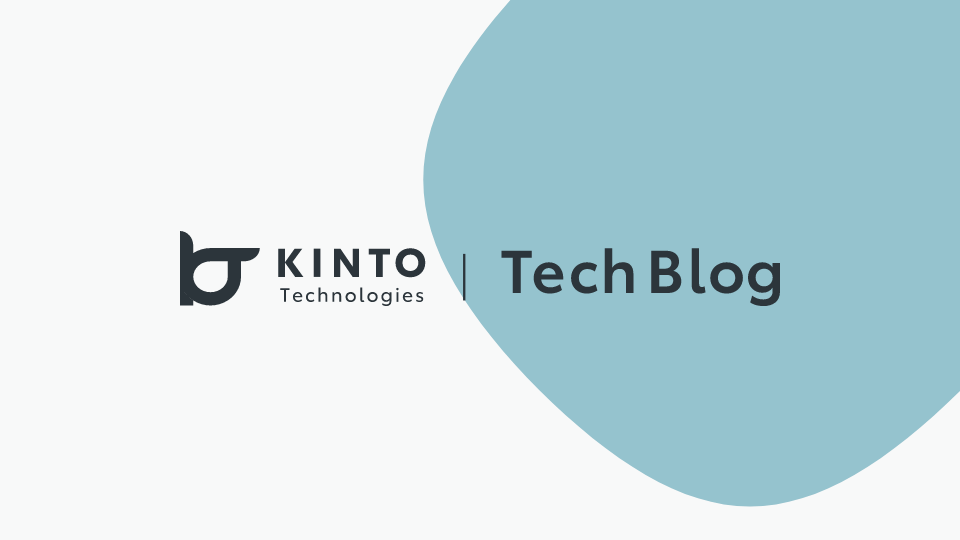
Build Your Front-end with Atomic Design!
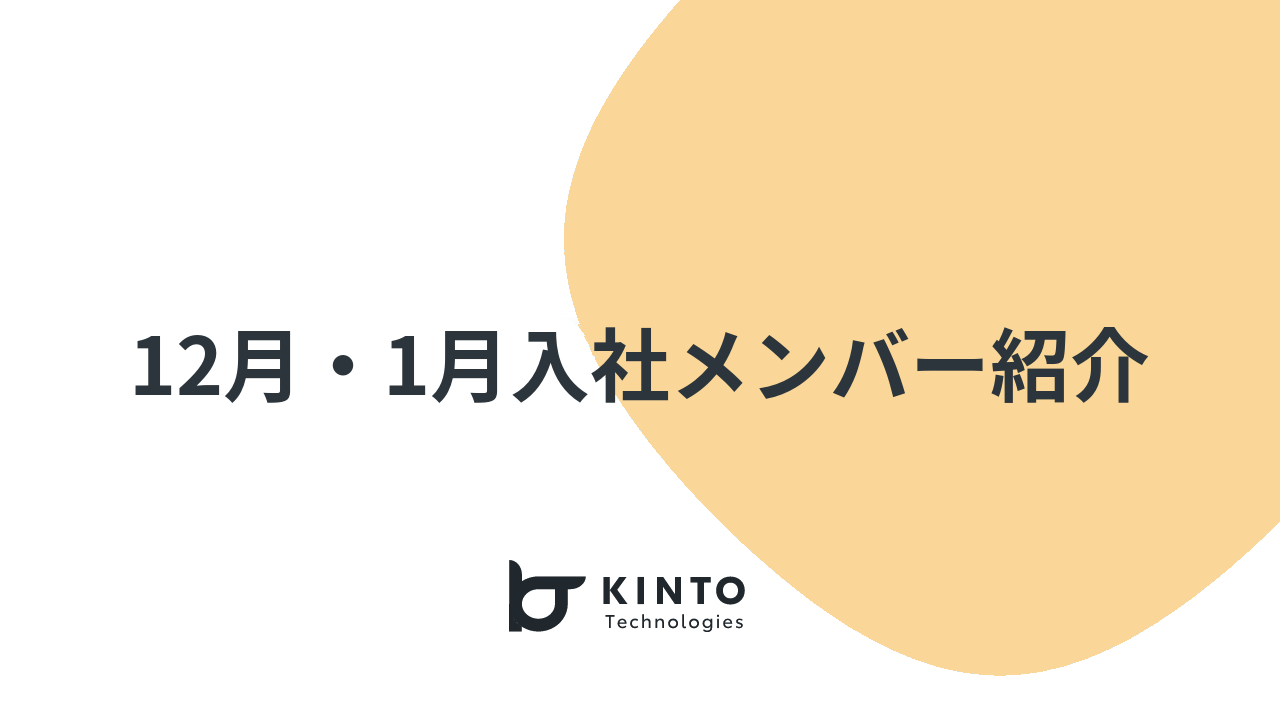
December and January Welcomes: Introducing the New Members
We are hiring!
【フロントエンドエンジニア】新車サブスク開発G/東京
新車サブスク開発グループについてTOYOTAのクルマのサブスクリプションサービスである『 KINTO ONE 』のWebサイトの開発、運用をしています。業務内容トヨタグループの金融、モビリティサービスの内製開発組織である同社にて、自社サービスである、TOYOTAのクルマのサブスクリプションサービス『KINTO ONE』のWebサイトの開発、運用を行っていただきます。
【フロントエンドエンジニア(コンテンツ開発)】新車サブスク開発G/東京
新車サブスク開発グループについてTOYOTAのクルマのサブスクリプションサービスである『 KINTO ONE 』のWebサイトの開発、運用をしています。業務内容トヨタグループの金融、モビリティサービスの内製開発組織である同社にて、自社サービスである、クルマのサブスクリプションサービス『KINTO ONE』のWebサイトコンテンツの開発・運用業務を担っていただきます。