Svelteと他JSフレームワークの比較 - Svelte不定期連載-01
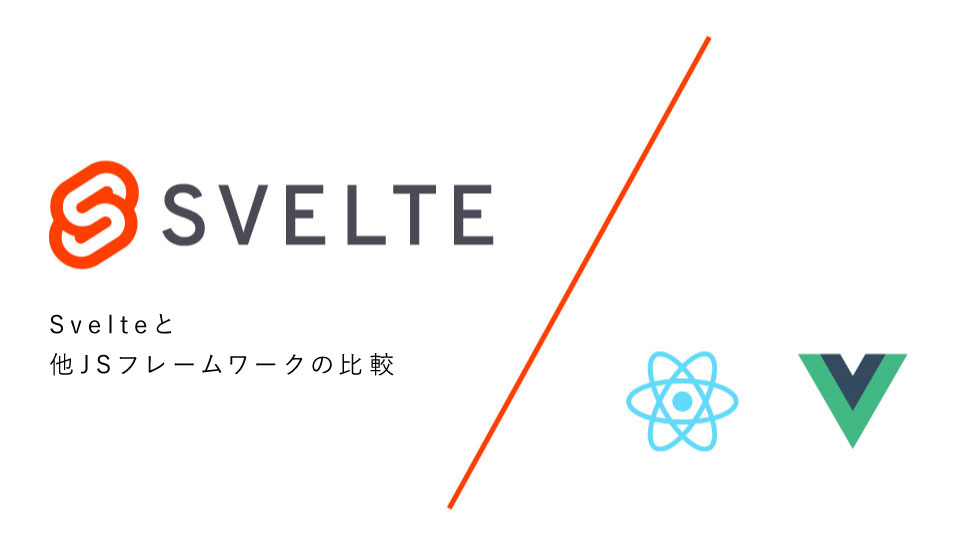
こんにちは(こんばんは)、始まりました。
Svelte不定期連載その1です。
前回はざっくりとSvelteKitを動かすまで、を書いてみました。
(SvelteKitのメジャーアップデートに伴って内容もアップデートしましたのでよかったら一読ください)
今回は、Write less codeをコンセプトとしたSvelteと他のJSフレームワークで、それぞれ書き方にどんな特徴があるのかを比較してみます。
Svelte / React / Vue.js
仮想DOMであるReactとVue.js、仮装DOMを持たないSvelteではありますが、比較対象としてはよくあげられるので改めて比較します。
※Svelte(v3系) / React(v18系) / Vue.js(v3系) で行います。
Fetch&ループ処理
Fetchとループ処理はセットで書かれることが多いので、まとめて記述してそれぞれを比較してみます。
Svelte
まずはSvelteから。
Svelteは独自の構文がありますね、
await/ block構文があるのですっきり書けるのが特徴です。
<script>
const fetchItems = async function() {
const items = await fetch('URL');
return await items.json();
}
</script>
{#await fetchItems()}
<p>Loading</p>
{:then items}
{#each items as item}
<p>{item.name}</p>
{/each}
{:catch error}
<p>{error.message}</p>
{/await}
React
Svelteと比べるとコードの行数が増えました。
とはいえ、本来であれば分割なりをするので、このまま書くことは滅多にないでしょう。
useStateではじめに定義するあたりがReactらしいのでしょうか。
function FetchComponent() {
const [error, setError] = useState(null)
const [isLoaded, setIsLoaded] = useState(false)
const [items, setItems] = useState([])
useEffect(() => {
fetch('URL')
.then(res => res.json())
.then(
(result) => {
setIsLoaded(true);
setItems(result)
},
(error) => {
setIsLoaded(true);
setError(error)
}
)
}, [])
if (!isLoaded) {
return <div>Error: {error.message}</div>;
} else if (!isLoaded) {
return <div>Loading...</div>;
} else {
return (
<ul>
{items.map(item => (
<li key={item.id}>
{item.name}
</li>
))}
</ul>
);
}
}
Vue.js
次はVue3、
2系と比べるとReactに書き心地が似てきた感覚があります。
<script setup>
import { ref } from 'vue'
const items = ref(null)
const error = ref(null)
fetch('URL')
.then((res) => res.json())
.then((json) => (items.value = json))
.catch((err) => (error.value = err))
</script>
<template>
<div v-if="error">{{ error.message }}</div>
<div v-else-if="items">
<ul v-for="(item,index) in items">
<li>Number:{{index+1}} Name:{{item.name}}</li>
</ul>
</div>
<div v-else>Loading</div>
</template>
Reactive
これもコンポーネントを使い回す現代のフロントエンドで必須の仕組みですね。
Svelte
Svelteでは接頭辞に$:
をつけることでリアクティブになります。
$:
をつけない限り依存する値が変更されても実行されません。
他のフレームワークになれているとこのあたりは最初面食らうかもしれません。
いざ使うと明示的だなぁと感動します。
$: foo = false
React
細かい説明を省きますが、ReactではuseStateで定義するのが一般的です。
Svelteと比較すると、useStateが $: と同等の役割でしょうか。
import {useState} from 'react'
const [foo] = useState(false)
Vue.js
Vueの場合はreactiveで生成すると変更されます。
<script setup>
import { reactive, computed } from 'vue'
const state = reactive({
count: 0
})
const increment = () => {
state.count++
}
</script>
propsについて
今回は基礎である文字列を受け渡す方法で比較してみます。
三者三様かと思いきや、ほとんど同じような書き心地になってきました。
Svelte
<script>
export let name
</script>
<p>Hello, I'm :{name}</p>
<script>
import ChildComponent from './ChildComponent.svelte'
</script>
<ChildComponent name="Svelte" />
React
const ChildComponent = (props) => {
return <h1>Hello, I'm :{props.name}</h1>;
}
export default ChildComponent;
import ChildComponent from './ChildComponent.jsx'
function App() {
return (
<ChildComponent name="React" />
);
}
export default App
Vue.js
<template>
<p>Hello, I'm {{name}}</p>
</template>
export default {
props: {
name: String,
}
}
<script>
import ChildComponent from './ChildComponent.vue'
</script>
<template>
<ChildComponent name="Vue" />
</template>
子コンポーネントをimportして値を渡す。
どれもわかりやすい書き方でした。
総評
短いですがSvelteと、React、Vue.jsを比較してみましたがいかがでしたでしょうか。
それぞれ良さがある中で、Write less codeをテーマにしたSvelte。
とっつきやすさがやはり際立っている印象があります。
よきSvelteライフをお過ごしください。
そして初めての方はSvelteを是非書いてみてください、とても楽しいフレームワークです。
関連記事 | Related Posts
We are hiring!
【フロントエンドエンジニア(コンテンツ開発)】新車サブスク開発G/大阪・福岡
新車サブスク開発グループについてTOYOTAのクルマのサブスクリプションサービスである『 KINTO ONE 』のWebサイトの開発、運用をしています。業務内容トヨタグループの金融、モビリティサービスの内製開発組織である同社にて、自社サービスである、クルマのサブスクリプションサービス『KINTO ONE』のWebサイトコンテンツの開発・運用業務を担っていただきます。
【フロントエンドエンジニア(リードクラス)】KINTO FACTORY開発G/東京
KINTO FACTORYについて自動車のソフトウェア、ハードウェア両面でのアップグレードを行う新サービスです。トヨタ/レクサス/GRの車をお持ちのお客様にOTAやハードウェアアップデートを通してリフォーム、アップグレード、パーソナライズなどを提供し購入後にも進化続ける自動車を提供するモビリティ業界における先端のサービスを提供します。